Use MAMP's PHP Version in Terminal
MAMP is a commercial server stack developed and sold by appsolute. On Mac OS its pretty much the best LAMP stack you can find. MAMP comes as a free version with limited functionality and a fee-based version (MAMP PRO). In MAMP PRO you have some additional features. For example you can easily change the used PHP version and you can also use this PHP version in the Terminal. But with a little script I wrote, this can also be accomplished with the free version.
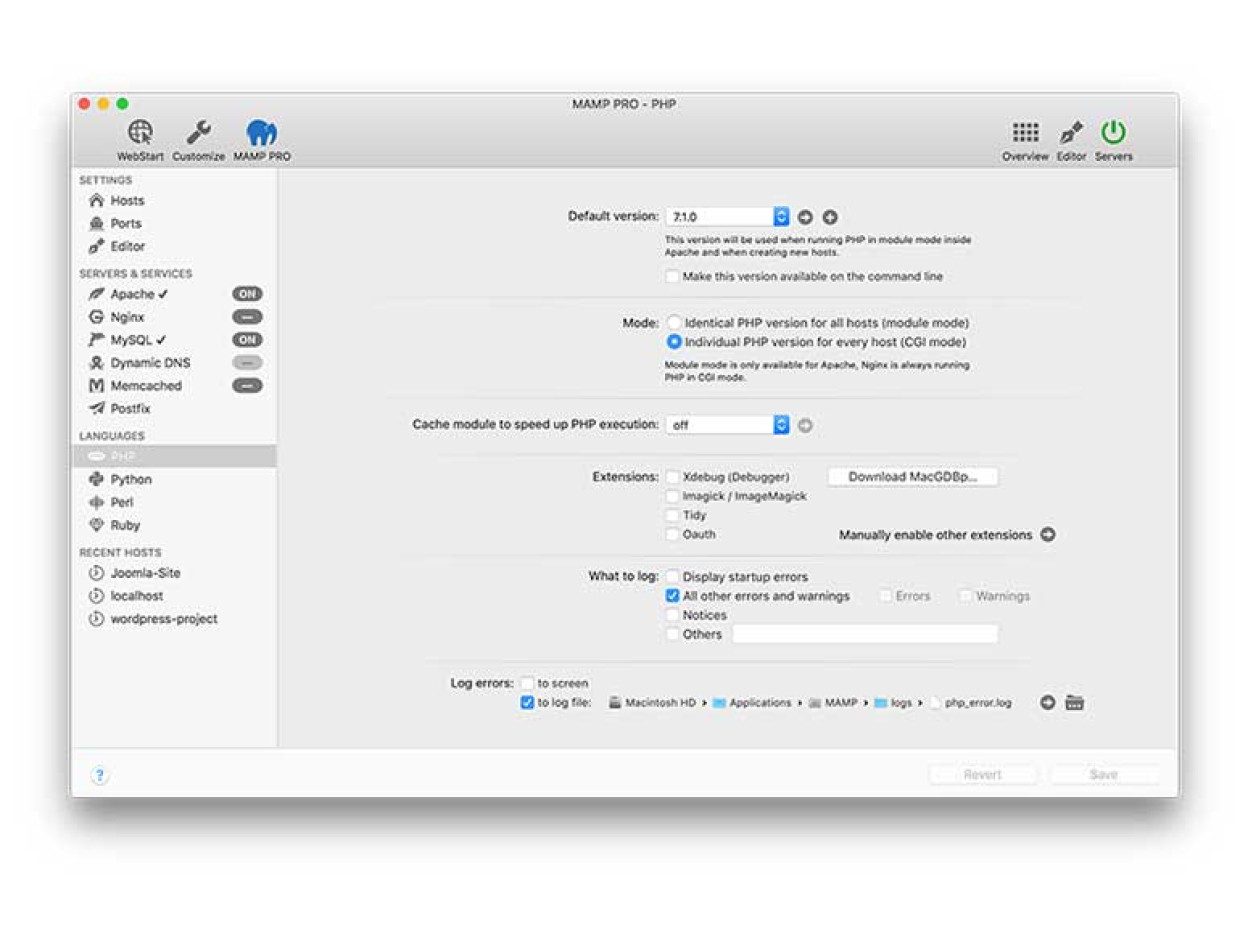
MAMPs installed PHP versions are located in a specific folder in your Applications directory. Using the free version, you can install new PHP versions by simply dropping them in there. On the most recent version this is /Applications/MAMP/bin/php/. So to determine which PHP versions are available I just needed to create a little Bash function, which scans the folder and outputs the directory names. Then I can programmatically create a symlink to the binaries in this folder and were are done.
But lets start from the beginning. First we will define two variables. One for the MAMP folder and one for our local binaries folder. This way we can change them more easily.
PHP_DIR="/Applications/MAMP/bin/php/"
BIN_DIR="/usr/local/bin/"
We can use PHP_DIR to scan the directory using an ls command. We will just grep the folders with php in the name and remove this string from the output to get a nice and clean list of available versions.
for f in $(ls $PHP_DIR | grep php)
do
echo ${f#"php"}
done
The output will look something like this:
5.3.9
5.4.45
5.5.38
5.6.30
7.0.20
7.1.6
Now that we know which versions are available, we can point our script to a specific version and create symlinks in our binaries directory. Please note that we will have to use sudo in the script or you will have to change your binaries folder to something else. Also I will just remove all binaries already in place.
version="7.0.20"
PHP_VERSION_PATH="${PHP_DIR}php${version}/bin/"
if [ ! -d "$PHP_VERSION_PATH" ]; then
echo "PHP version $version does not exist."
exit 1
fi
# Ask for permissions right away
sudo -v
# Symlink all relevant binaries.
sudo rm -f "${BIN_DIR}php" "${BIN_DIR}pear" "${BIN_DIR}pecl"
sudo ln -s "${PHP_VERSION_PATH}php" "${BIN_DIR}php"
sudo ln -s "${PHP_VERSION_PATH}pear" "${BIN_DIR}pear"
sudo ln -s "${PHP_VERSION_PATH}pecl" "${BIN_DIR}pecl"
We will create symlinks for php, pear and pecl. Afterwards we should output the updated PHP version. Here I assume, that you have a .bash_profile file and use it to refresh your Terminal session. If you use something else, feel free to change it to your needs.
echo "Now using the following php version:"
. ~/.bash_profile
php -v
Of course now you just have a few lines of Bash code with no real context whatsoever. In the final script I created two functions and a little bit of business logic to map calls to the script to the correct function. You can get the script here or copy the contents of the code section below.
#!/bin/bash
PHP_DIR="/Applications/MAMP/bin/php/"
BIN_DIR="/usr/local/bin/"
function use_version {
PHP_VERSION_PATH="${PHP_DIR}php${1}/bin/"
if [ ! -d "$PHP_VERSION_PATH" ]; then
echo "PHP version $1 does not exist."
exit 1
fi
# Ask for permissions right away
sudo -v
# Symlink all relevant binaries.
sudo rm -f "${BIN_DIR}php" "${BIN_DIR}pear" "${BIN_DIR}pecl"
sudo ln -s "${PHP_VERSION_PATH}php" "${BIN_DIR}php"
sudo ln -s "${PHP_VERSION_PATH}pear" "${BIN_DIR}pear"
sudo ln -s "${PHP_VERSION_PATH}pecl" "${BIN_DIR}pecl"
# Output the now active php version.
echo "Now using the following php version:"
. ~/.bash_profile
php -v
exit
}
function list_versions {
for f in $(ls $PHP_DIR | grep php)
do
echo ${f#"php"}
done
}
case "$1" in
use)
use_version $2
;;
list)
list_versions
;;
*)
echo "Usage: $0 {list|use version}"
esac
exit 0
The installation is pretty simple. Download the script from the link above or create a file phpcv with the contents from the last code section. Then move the file to your local binaries folder and make it executable.
wget -O phpcv https://gist.githubusercontent.com/aureolacodes/dbed3f9848908774ad2eb4cc34f5b2fe/raw/3d887dfbcfbc2326c6e04e8de1f80732ffec4610/phpcv
chmod +x phpcv
sudo mv phpcv /usr/local/bin/phpcv
Run phpcv to view the available commands.
Usage: /usr/local/bin/phpcv {list|use version}
The script works with MAMP and MAMP PRO. Please note, that you shouldn't use this in combination with MAMP's "Make this version available on the command line" turned on. You might get some unexpected results. By the way... a simpler, but less flexible way would be to add the folder of the PHP version you need to your PATH variable.
export PATH="$PATH:/Applications/MAMP/bin/php/php7.0.20/bin"
There are no comments yet.