Capslock Detection via JavaScript
DO YOU KNOW THAT FEELING? YOU JUST TYPED IN YOUR USER CREDENTIALS AND INSTEAD OF LOGGING IN, YOU JUST GET AN ERROR MESSAGE. YOU WONDER IF YOU HAD A TYPO AND THAN YOU REALIZE THAT THIS RELICT OF ANCIENT TIMES SCREWED YOU OVER AGAIN... CAPS LOCK. To prevent this from happening to your users you can provide a little hint to your login forms. This article tells you how to do this in JavaScript, with and without jQuery.
The Browser as a Role Model
There are some browsers and operating systems which actually provide users with hints whenever caps lock is active. Chrome for example displays a little icon near the right edge of the password field. But users with less user-friendly browsers should not be left in the dark.
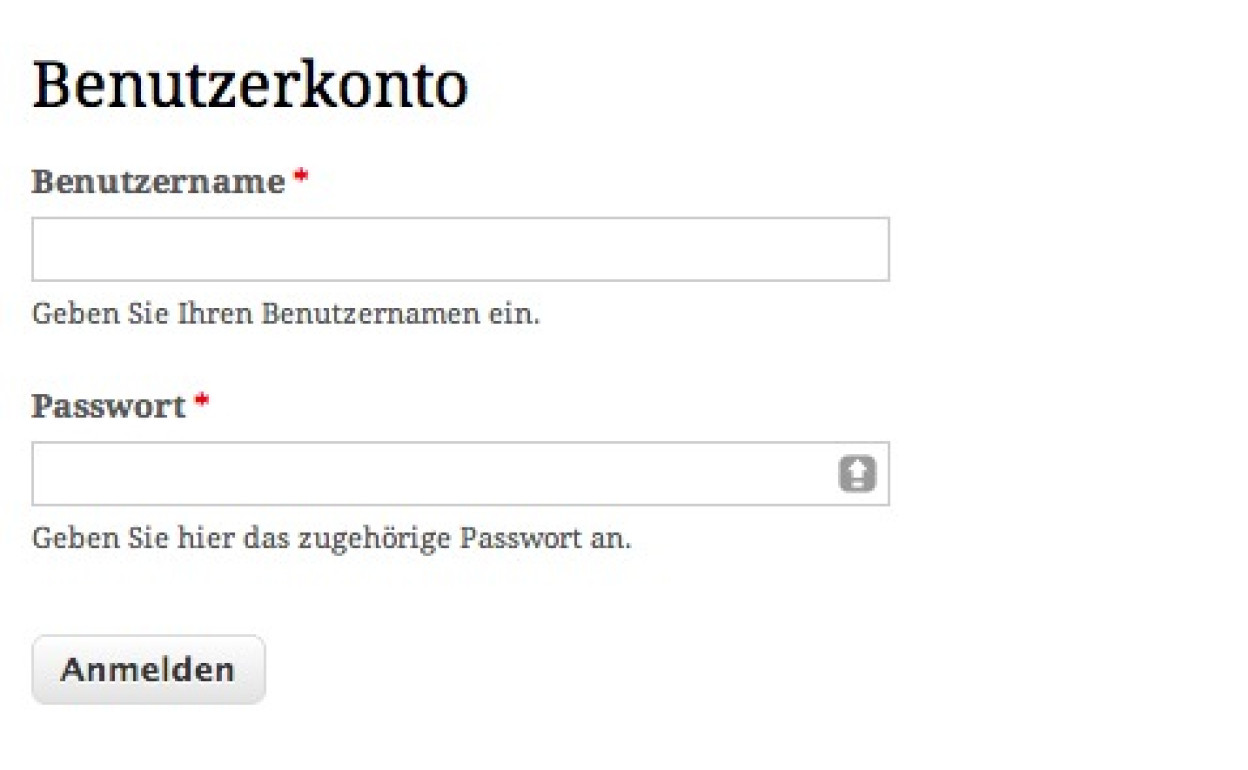
Key-Events to the Rescue
Before we begin we have to face an inconvenient truth. An activated caps lock key can only be determined by using key events (e.g. keypress). There is no other method to do this in JavaScript. But it should be noted that there are plugins like capslockstate which track the state of the caps lock key. In this article I will show you a simpler solution.
First let's define a simple password field:
<input type="password" name="myPassword" onkeypress="checkCapslock(event)">
Even with key events there is no direct way to check if caps lock is active. Instead we will check if the character added by the user is uppercase and the shift key was not pressed. In this case we can be certain, that caps lock has to be active. So we will just add a class capslock to the password field. If caps lock is not active, we will remove the class.
function checkCapslock(e) {
var c = String.fromCharCode(e.which),
capslockActive = (c.toUpperCase() === c && c.toLowerCase() !== c && !e.shiftKey);
if (capslockActive) {
$(this).addClass('capslock');
}
else {
$(this).removeClass('capslock');
}
}
And now without jQuery
You don't want to use jQuery? No problem. The following code does the exact same thing without it.
function checkCapslock(e) {
var c = String.fromCharCode(e.which),
capslockActive = (c.toUpperCase() === c && c.toLowerCase() !== c && !e.shiftKey);
if (capslockActive && e.target.className.indexOf('capslock') === -1) {
e.target.className += ' capslock';
}
else if (!capslockActive && e.target.className.indexOf('capslock') !== -1) {
e.target.className = e.target.className.replace(' capslock', '');
}
}
Discreet Hints
We can use the class capslock to add styles to the password field in case caps lock is active. For example we could just turn it red...
.capslock { background: red; }
In real life you will probably want to add a more obvious hint, like the icon used by Chrome. Have fun trying it out. You might also want to check the following links for more information on the subject.
There are no comments yet.