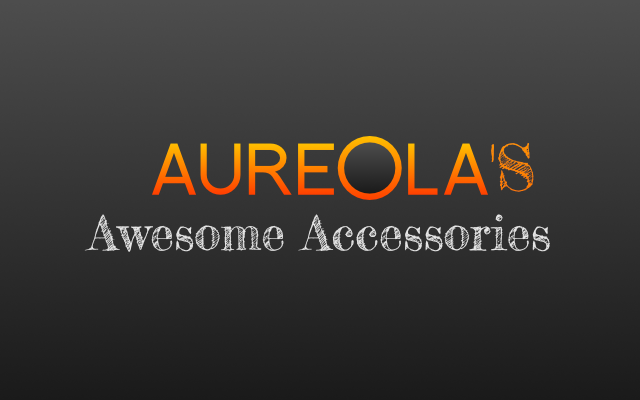
I've been involved with Unity and game development for a number of years. Over the years, I have come up with many solutions to problems that you inevitably face as a game developer. Where do I store user preferences? How do I handle translations? How can I easily play sounds without having to write a custom solution every time? For all these problems and more, I have found solutions over time and used them in all my projects. And I would like to share these solutions with you.
This Unity packages includes all these little helpers, services and time savers. The package is available on Github and maybe later released to the Unity Asset Store. It will always be open source and is released under the MIT license. It includes solutions for UI sounds, configuration management, loading scenes, event management, user preferences, data storage, translations and more.
These custom solutions have made my life as a game developer easier for years, and I hope they will help you too. I look forward to your feedback.
Core Concepts
In order to make this collection of utilities more accessible, I have defined some core concepts that I adhere to when developing this package.
Managers
Managers are the core of this package. They are responsible for managing a specific problem domain, such as the AudioManager
for handling audio playback or the TranslationManager
for handling translations. All managers are Scriptable Objects and can be added to every behaviour in your project. You can have multiple instances of a manager, but you should only have one instance of a manager per behaviour.
Behaviours
Behaviours are the core of Unity. They are the building blocks of every game or application. This package provides a set of behaviours that can be used to extend the functionality of Unity's built-in behaviours. For example, the PlayGlobalSound
can be used to play audio clips in a more convenient way.
Communication & Delegates
Managers and Behaviours communicate with each other using delegates. This allows for a more decoupled architecture and makes it easier to extend the functionality of the package.
Services
Services are used to provide additional functionality used by certain managers or behaviours. For example, the FilesService
is used to read and write files to the file system. You need to instantiate services manually inside the behaviour or manager that uses them.
Modules
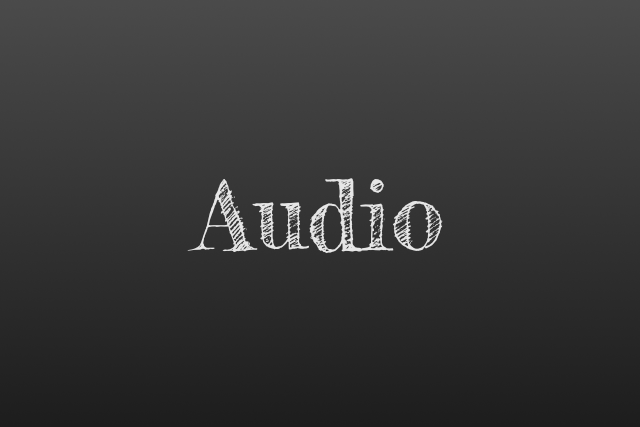
The AudioManager is designed to manage audio sources and volumes within a Unity project. It allows for the control of music, sound effects, and voice separately with individual volume controls as well as a master volume control that affects all audio sources. The class includes functionality to play, stop, and check if audio is playing. It also provides adjusted volume properties that take into account both individual and master volumes.
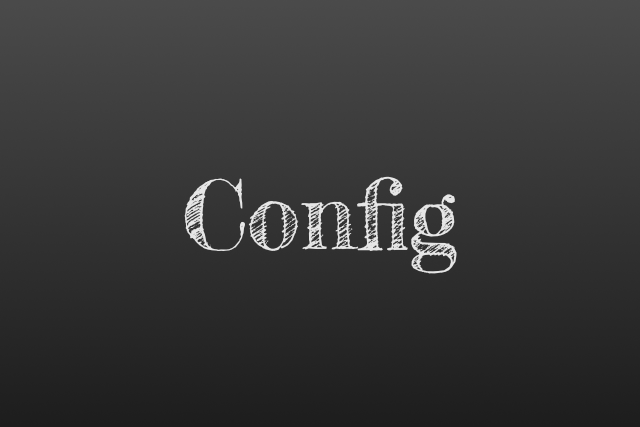
The ConfigManager is designed to manage configuration settings for a Unity project. It loads configuration data from a specified JSON file and stores the data in a runtime cache. The class supports a variety of data types, including primitives, Vector2, Vector3, Quaternion, and Color. It also includes functionality to clear the cache and retrieve stored values.
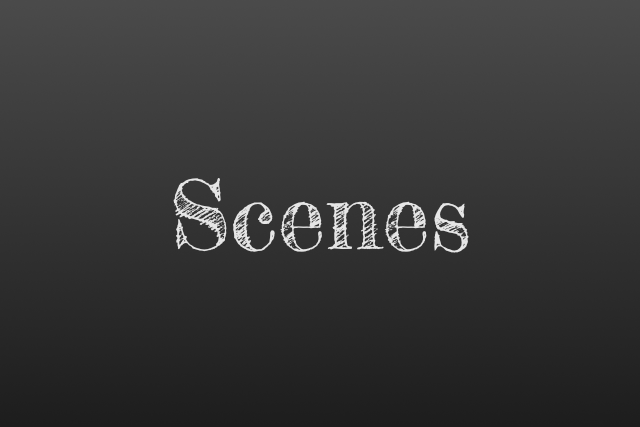
The ScenesManager is designed to manage scene operations such as loading, exiting, and transitioning between scenes in a Unity project. It allows for asynchronous scene loading and unloading, with support for additive scenes. The class also provides events for scene operations, enabling custom behaviors during scene transitions.
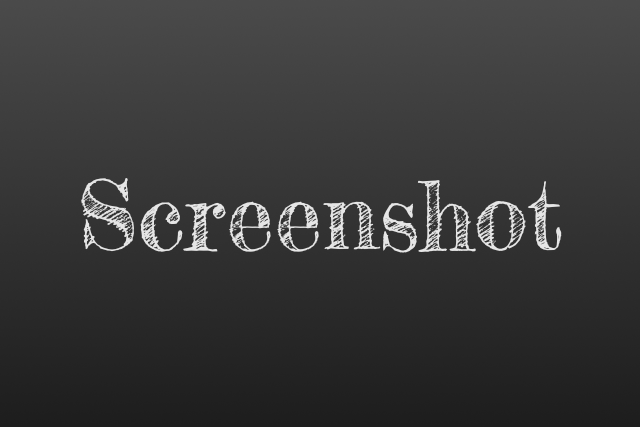
The ScreenshotManager facilitates the capture and storage of screenshots within a Unity project. It allows customization of the storage directory and includes optional debug logging. This class utilizes a coroutine to capture screenshots at the end of the current frame, ensuring that the captured image represents the fully rendered scene.
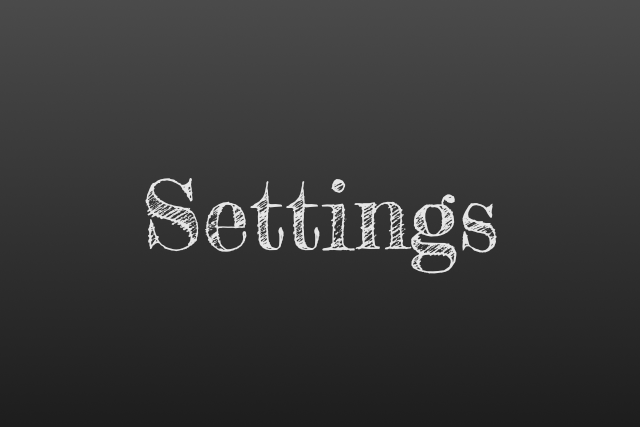
The SettingsManager facilitates the management of application settings within a Unity project. It provides a structure for loading, saving, and accessing settings data through a customizable settings driver. The class raises events on key operations such as loading, saving, changes, and errors, allowing for responsive and dynamic settings management.
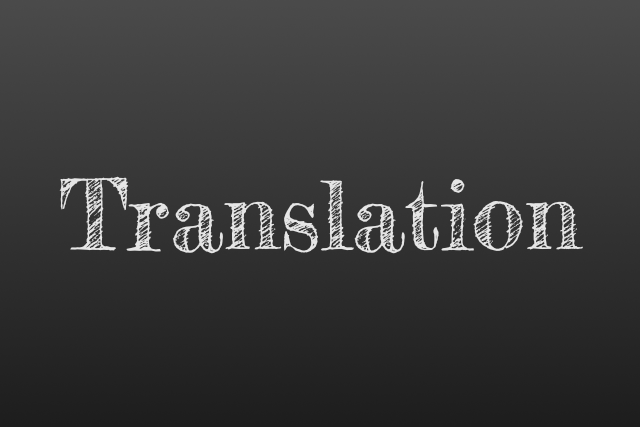
The TranslationsManager is designed to facilitate the management of translations within a Unity application, providing the infrastructure to support multiple languages. It allows for dynamic language switching, supports the retrieval of translated strings, and manages the active translation set.
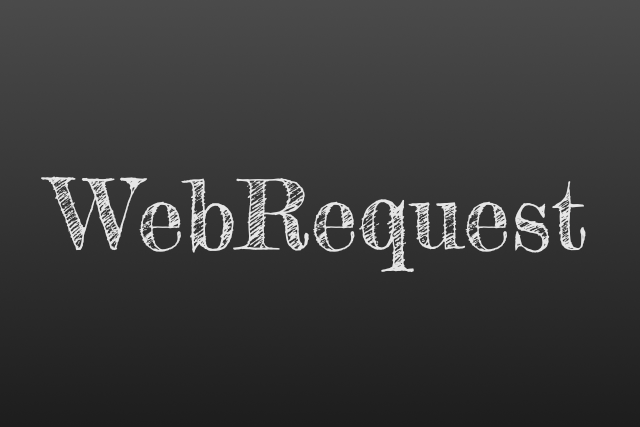
The WebRequestService provides a flexible and convenient way to handle web requests within Unity applications. It supports GET, POST, and other HTTP methods, customizable timeouts, debugging options, and callbacks for success and failure scenarios.
Support the Project
If you find this project helpful and would like to contribute to its development and ongoing maintenance, your support would be greatly appreciated. By making a donation, you can help ensure the sustainability of the project and allow me to devote more time and resources to improving it.
How can you contribute?
Financial contributions: If you would like to make a monetary donation, you can do so securely through PayPal. Every contribution, no matter the amount, makes a difference and is greatly appreciated.
Bug Reports and Feature Requests: If you encounter problems while using the project, or have ideas for new features, please open an issue in the GitHub repository. Your feedback and suggestions are critical to improving the project for everyone.
Code Contributions: If you are a developer and would like to contribute directly to the project's codebase, feel free to submit a pull request. Contributions of all sizes are welcome and will be acknowledged.
Your Contribution Counts, No Matter the Form
Even if you are unable to contribute financially or through code, there are other ways to show your support:
Star the project on GitHub. This will help increase its visibility and attract more users.
Share the project with others. Spread the word about the project and help it reach a wider audience.
Your support means a lot to me, and it motivates me to keep working on and improving this project. Thank you for considering a donation and supporting its development!
Support
This package should work in all currently supported Unity versions. If you have any questions or need help with the package, join the Discord Server or open an issue on GitHub.
License
MIT License, Copyright (c) 2023 Christian Hanne